반응형
목표
- 딥러닝 신경망으로 iris 다중분류 문제를 해결해보자
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
iris = load_iris()
iris.keys()
dict_keys(['data', 'target', 'frame', 'target_names', 'DESCR', 'feature_names', 'filename', 'data_module'])
iris.feature_names
iris.feature_names
['sepal length (cm)',
'sepal width (cm)',
'petal length (cm)',
'petal width (cm)']
X = iris['data']
y = iris['target']
X.shape, y.shape
((150, 4), (150,))
y #정답 3가지(0,1,2) -> 다중분류
array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2])
# 같은 스케일로 오차를 구해주기 위해서 정답데이터를 원핫인코딩 시킨다
y_one_hot = pd.get_dummies(y)
y_one_hot
0 1 2
0 1 0 0
1 1 0 0
2 1 0 0
3 1 0 0
4 1 0 0
... ... ... ...
145 0 0 1
146 0 0 1
147 0 0 1
148 0 0 1
149 0 0 1
150 rows × 3 columns
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X,y_one_hot,
test_size=0.2,
random_state=3)
print(X_train.shape)
print(X_test.shape)
print(y_train.shape)
print(y_test.shape)
(120, 4)
(30, 4)
(120, 3)
(30, 3)
신경망 모델링
-1.신경망 구조 설계
-2. 학습/평가방법 설정
-3. 학습 및 시각화
-4. 모델평가
- 출력층 뉴런의 개수는 실제 정답의 개수만큼 설정
- 출력층 활성화함수 softmax
- 손실함수 categorical_crossentropy
from tensorflow.keras import Sequential
from tensorflow.keras.layers import Dense
model = Sequential()
# 입력층 + 중간층 (X_train의 특성 개수를 입력)
model.add(Dense(100, input_dim=4, activation="relu"))
model.add(Dense(50, activation="relu"))
model.add(Dense(30, activation="relu"))
model.add(Dense(10, activation="relu"))
# 출력층 뉴런의 개수는 원핫인코딩 된 컬럼 개수
model.add(Dense(3, activation="softmax"))
model.compile(loss="categorical_crossentropy",
optimizer="Adam",
metrics=['acc'])
h= model.fit(X_train,y_train, epochs=100)
plt.figure(figsize=(15,5))
plt.plot(h.history['acc'], label='acc')
plt.legend()
plt.show()
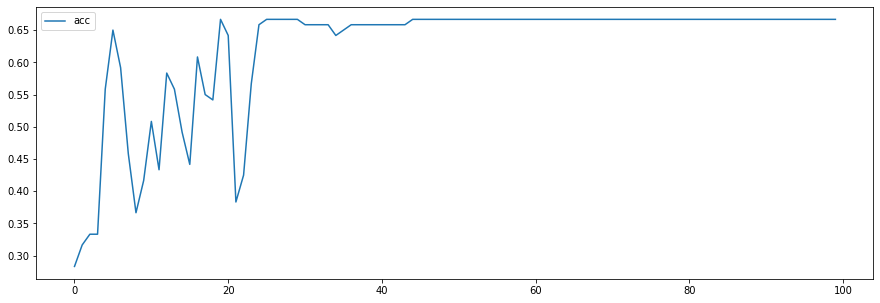
plt.figure(figsize=(15,5))
plt.plot(h.history['loss'], label='loss')
plt.legend()
plt.show()
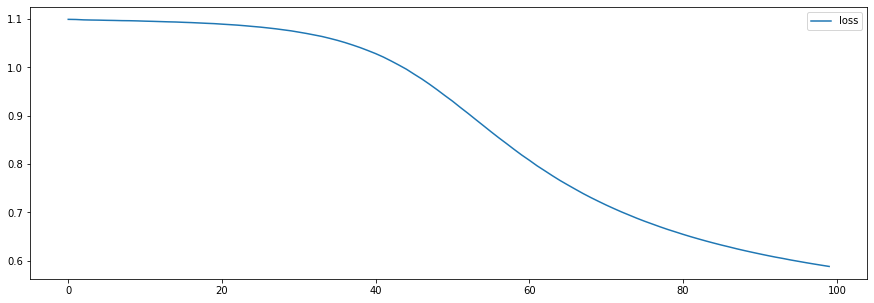
model.evaluate(X_test,y_test)
1/1 [==============================] - 0s 156ms/step - loss: 0.5865 - acc: 0.6667
[0.5864841938018799, 0.6666666865348816]
model.predict(X_test)
원핫 인코딩결과 0,0 이 답일때 답일 확률 -> 0.84 (0,0)/ 0.059(0,1) / 0.093(0,2) 와 매칭
1. 회귀
- loss = mse(평균제곱오차)
- 출력층 뉴련 개수 : 1개
- 출력층 활성화함수 : linear(디폴트값, 생략가능)
2. 이진분류
- loss = binary_crossentropy
- 출력층 뉴련 개수 : 1개
- 출력층 활성화함수 : sigmoid
3. 다중분류
- loss = categorical_crossentropy
- 출력층 뉴련 개수 : 정답의 개수(원핫인코딩된 특성 수)
- 출력층 활성화함수 : softmax
반응형
'빅데이터 서비스 교육 > 딥러닝' 카테고리의 다른 글
사람 얼굴 이진분류 모델 생성 (0) | 2022.07.19 |
---|---|
이미지 데이터 분류 (0) | 2022.07.18 |
활성화함수 (0) | 2022.07.14 |
딥러닝 유방암 데이터 분류 예제 (0) | 2022.07.14 |
딥러닝 2진분류 실습 (폐암환자 예측) (0) | 2022.07.13 |